what is more on uno r3 development module board
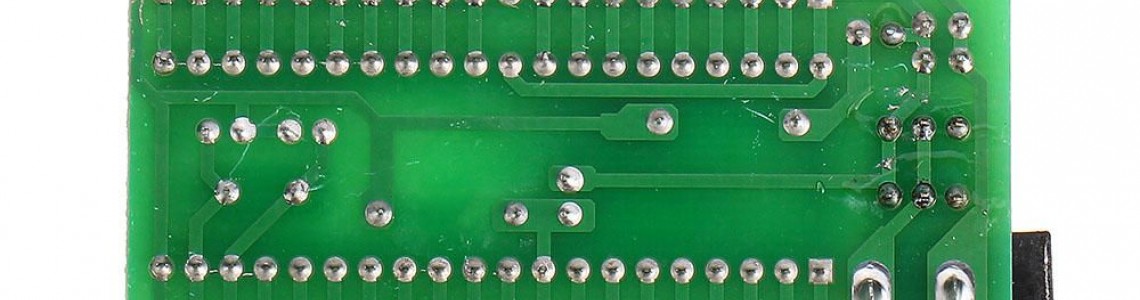
2.1 startup process
generally speaking, our c language program starts with a main function, but in the previous tutorial we found that there are only two functions, setup and loop, in the file generated by the ide, so how does arduino call them?
in fact, the real main function lives in our arduino library file (located in arduino->main.cpp), which is defined as follows:
int main(void)
{
// do some hardware and variable initialization work
init();
initvariant();
#if defined(usbcon)
usbdevice.attach();
#endif
// call the setup() function we wrote
setup() ;
for (;;) {
// call the loop() function we wrote
loop();
if (serialeventrun) serialeventrun();
}
return 0;
}
you can see that the two functions we wrote setup and loop will be called in main. of course, how the related files are organized and compiled is the function provided by the arduino tool chain. we will not do in-depth understanding here. in the beginner stage, we only care about how to use it.
2.2 commonly used functions
arduino provides a variety of functions for us to use, the details can be found in the arduino api manual.
don't memorize related functions, make good use of ide's intelligent completion and search engine, you can get started quickly
next, we briefly introduce several commonly used functions by explaining the above lighting code:
void setup(): initialize related pins and variables
when the program runs in arduino, the setup() function will be called first , which is used to initialize variables, set the output/input type of pins, configure serial ports, import class library files, and so on. the setup function only runs once each time the arduino is powered up or restarted, for example:
void setup()
{
pinmode(led_builtin, output); // set the built-in led port to output mode
}
after that, the loop() function will be executed. as the name implies, this function will loop continuously during the running of the program until the chip is powered off.
void loop()
{
delay(300); // wait for 300ms
digitalwrite(led_builtin, high);// built-in led output high level, turn on the light
delay(300);
digitalwrite(led_builtin, low);// built-in led output low level, off, etc.
}
the code in the loop turns the lights on/off every 300ms to achieve the effect of flashing lights. the following is a detailed explanation of the constants and functions used:
constant
high | low: indicates the level of the digital io port, high indicates a high level (1 means the output voltage is "lit"), low means a low level (0 means no output voltage "off")
input | output: indicates the direction of the digital io port, input indicates the input (high-impedance state, that is, the input voltage signal can be read as if the resistance is very large), and output indicates (the output voltage signal)
digital i/o
pinmode(pin, mode): the definition function of the input and output mode of the digital io port, the parameter mode can be input or output
digitalwrite(pin, value): the function of defining the output level of the digital io port, the parameter value can be high or low, which can be used to light up the led
int digitalread(pin): digital io port read input level function, the return value is high or low, which can be used to read digital sensors
note: the range of parameter pin value is 0~13, which refers to 14 pins.
time function
delay(ms): delay function (unit ms)
the above are common functions, you don’t need to remember to have an impression, and you will remember them when you use them frequently later.
Leave a Comment